In this tutorial we will learn a Java program to create an array and rotate the elements stored in the array by two positions.
Our program will first take the input of the array size and then the elements of the array. Then our program will rotate the elements of the array by double
For example
Case 1: If the user enters 4 as the array (list) size and the array (list) elements as 1,2,3,4, the output should be 3, 4, 1, 2.
Case 2: If the user enters 5 as the array (list) size and the array (list) elements as 9, 8, 7, 6, 5, the output should be 6, 5, 9, 8, 7.
Right Rotation on Array Elements by Two Position in Java
import java.util.*;
public class ArrayExample {
public static void main(String[] args) {
int temp;
Scanner sc = new Scanner(System.in);
System.out.println("Java Program to perform right rotation two times");
System.out.print("Enter the size of array: ");
int size = sc.nextInt();
int arr[] = new int[size];
for (int i = 0; i < size; i++) {
System.out.print("Please give value for index " + i + " : ");
arr[i] = sc.nextInt();
}
for (int i = 0; i < 2; i++) {
temp = arr[size - 1];
for (int j = size - 1; j > 0; j--) {
arr[j] = arr[j - 1];
}
arr[0] = temp;
}
System.out.println("Array after two time right rotation");
for (int i = 0; i < size; i++)
{
System.out.print(arr[i] + "\t");
}
}
}
Explanation
For the input field: [1, 2, 3, 4, 5], the for loop will rotate the array 2 times.
On the 1st rotation, the array becomes: [5, 1, 2, 3, 4].
And on the 2nd rotation, the array will finally become: [4, 5, 1, 2, 3]
That means if our array (list) is
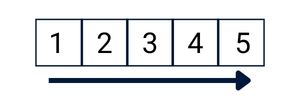
After two rotations
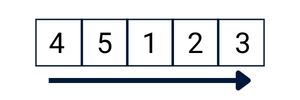
Output
Java Program to perform right rotation two times
Enter the size of array: 5
Please give value for index 0 : 1
Please give value for index 1 : 2
Please give value for index 2 : 3
Please give value for index 3 : 4
Please give value for index 4 : 5
Array after two time right rotation
4 5 1 2 3