In this tutorial, we will learn writing java program to create an array and perform left rotation on elements stored in the array by two positions.
Perform Left Rotation on Array Elements by Twice
import java.util.*;
public class ArrayExample {
public static void main(String[] args) {
int temp;
Scanner sc = new Scanner(System.in);
System.out.println("Java Program to perform right rotation two times");
System.out.print("Enter the size of array: ");
int size = sc.nextInt();
int arr[] = new int[size];
for (int i = 0; i < size; i++) {
System.out.print("Please give value for index " + i + " : ");
arr[i] = sc.nextInt();
}
for (int i = 0; i < 2; i++) {
temp = arr[0];
for (int j = 0; j < size - 1; j++) {
arr[j] = arr[j + 1];
}
arr[size - 1] = temp;
}
System.out.println("Array after two time right rotation");
for (int i = 0; i < size; i++) {
System.out.print(arr[i] + "\t");
}
}
}
Explanation
For the input array: [1, 2, 3, 4, 5], the for loop will rotate the array 2 times: [2, 3, 4, 5, 1] and finally for the second rotation, the array becomes [3, 4, 5, 1, 2]: [3, 4, 5, 1, 2]
That means if our array (list) is
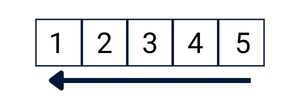
After two left rotations
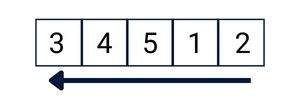
Output
Java Program to perform right rotation two times
Enter the size of array: 5
Please give value for index 0 : 1
Please give value for index 1 : 2
Please give value for index 2 : 3
Please give value for index 3 : 4
Please give value for index 4 : 5
Array after two time right rotation
3 4 5 1 2