In this tutorial we are going to learn writing java program to calculate the Factorial of a given number using recursion.
What is Recursion?
Recursion is a method that defines something in terms of itself, which means it is a process in which a function calls itself again and again till condition satisfy.
A complete function can be split down into various sub-parts in recursion. It makes code look simple and compact. It is also defined as the process of defining a program or problem in terms of itself. Let’s have a look at the simple diagram that explains the working of recursion.
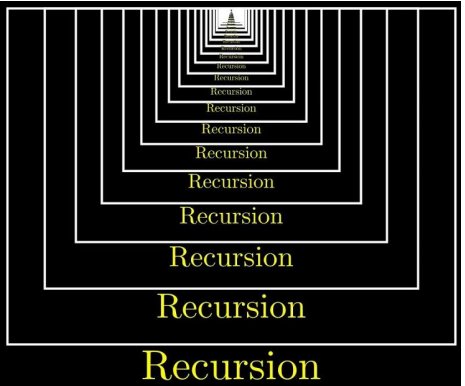
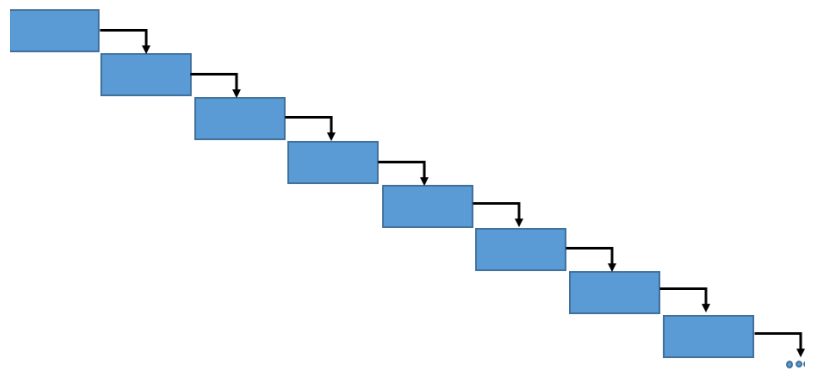
Our logic of Factorial program using recursion
- Our program will take an integer input from the user which should be a whole number.
- If the number input is negative then print ‘The factorial can’t be calculated’.
- Then use fact(num) user-defined recursive function that will return the factorial of the given number.
Let’s understand through a diagram how our recursive function will work on input 7.
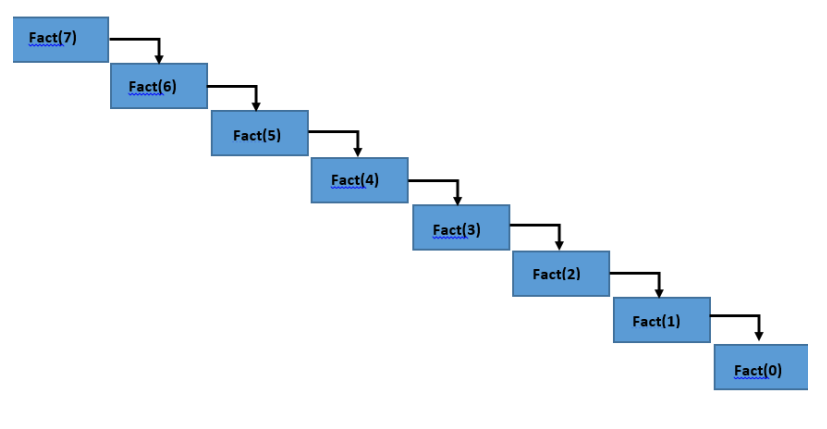
Algorithm to calculate factorial using recursion
Step 1: Start
Step 2: Create a user-define function ‘fact(num)’ which take an integer as an parameter and return num*fact(num-1).
Step 3: Create a variable num.
Step 4: Take an integer input from the user and store it into num variable.
Step 5: Pass the variable num as the parameter of user-defined function ‘func’ and print the result returned by the function.
Step 6: Stop
Java Program to calculate factorial using recursion
import java.util.*;
public class Main {
static long fact(long num){
if (num == 0){
return 1;
} else {
return(num * fact(num-1));
}
}
public static void main(String[] args) {
long num;
Scanner sc = new Scanner(System.in);
System.out.println("Enter a whole number to find Factorial ");
num= sc.nextInt();
System.out.println("Factorial = "+fact(num));
}
}
Output:
Enter a whole number to find Factorial
5
Factorial = 120
Explanation
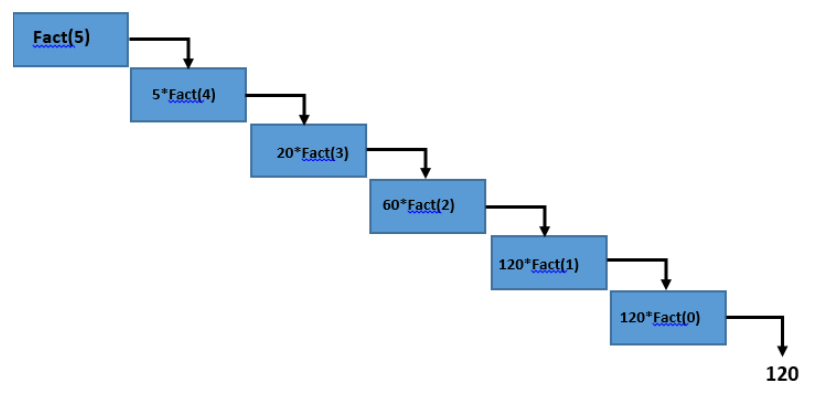